This script creates a graphical Java application. Using this application user will be able to perform some analysis on text. The analysis will allow the user to check if the data entered is a palindrome and will also report several other statistics of the usage of the characters that comprises the entered data.
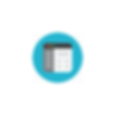
import java.awt.Color;
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
/**
*
* @author danielwevers
*/
public class PalindromeChecker extends javax.swing.JFrame {
/**
* Creates new form PalindromeChecker
*/
public PalindromeChecker() {
initComponents();
}
/**
* This method is called from within the constructor to initialize the form.
* WARNING: Do NOT modify this code. The content of this method is always
* regenerated by the Form Editor.
*/
@SuppressWarnings("unchecked")
// <editor-fold defaultstate="collapsed" desc="Generated Code">//GEN-BEGIN:initComponents
private void initComponents() {
jTextField1 = new javax.swing.JTextField();
jButton1 = new javax.swing.JButton();
jLabel1 = new javax.swing.JLabel();
jPanel1 = new javax.swing.JPanel();
jLabel8 = new javax.swing.JLabel();
jLabel9 = new javax.swing.JLabel();
jLabel10 = new javax.swing.JLabel();
jLabel11 = new javax.swing.JLabel();
jLabel12 = new javax.swing.JLabel();
jLabel13 = new javax.swing.JLabel();
jLabel2 = new javax.swing.JLabel();
jLabel3 = new javax.swing.JLabel();
jLabel4 = new javax.swing.JLabel();
jLabel5 = new javax.swing.JLabel();
jLabel6 = new javax.swing.JLabel();
jLabel7 = new javax.swing.JLabel();
jLabel14 = new javax.swing.JLabel();
jLabel15 = new javax.swing.JLabel();
setDefaultCloseOperation(javax.swing.WindowConstants.EXIT_ON_CLOSE);
jButton1.setText("Check");
jButton1.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
jButton1ActionPerformed(evt);
}
});
jLabel1.setFont(new java.awt.Font("Lucida Grande", 0, 24)); // NOI18N
jLabel1.setText("Is it a Palindrome?");
jPanel1.setBackground(java.awt.Color.white);
jLabel8.setText("0");
jLabel9.setText("0");
jLabel10.setText("0");
jLabel11.setText("0");
jLabel12.setText("0");
jLabel13.setText("0");
jLabel2.setText("Alpha:");
jLabel3.setText("Num:");
jLabel4.setText("Control: ");
jLabel5.setText("Upper: ");
jLabel6.setText("Lower:");
jLabel7.setText("White Space:");
javax.swing.GroupLayout jPanel1Layout = new javax.swing.GroupLayout(jPanel1);
jPanel1.setLayout(jPanel1Layout);
jPanel1Layout.setHorizontalGroup(
jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(javax.swing.GroupLayout.Alignment.TRAILING, jPanel1Layout.createSequentialGroup()
.addContainerGap(30, Short.MAX_VALUE)
.addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel1Layout.createSequentialGroup()
.addComponent(jLabel5)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel11)
.addGap(20, 20, 20)
.addComponent(jLabel6)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel12)
.addGap(43, 43, 43)
.addComponent(jLabel7)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel13))
.addGroup(jPanel1Layout.createSequentialGroup()
.addComponent(jLabel2)
.addGap(11, 11, 11)
.addComponent(jLabel8)
.addGap(20, 20, 20)
.addComponent(jLabel3)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel9)
.addGap(50, 50, 50)
.addComponent(jLabel4)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel10)))
.addGap(16, 16, 16))
);
jPanel1Layout.setVerticalGroup(
jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel1Layout.createSequentialGroup()
.addGap(25, 25, 25)
.addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jLabel2, javax.swing.GroupLayout.Alignment.TRAILING)
.addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(jLabel4)
.addComponent(jLabel3)
.addComponent(jLabel8)
.addComponent(jLabel9)
.addComponent(jLabel10)))
.addGap(18, 18, 18)
.addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(jLabel5)
.addComponent(jLabel6)
.addComponent(jLabel7)
.addComponent(jLabel11)
.addComponent(jLabel12)
.addComponent(jLabel13))
.addContainerGap(25, Short.MAX_VALUE))
);
jLabel14.setFont(new java.awt.Font("Lucida Grande", 1, 18)); // NOI18N
jLabel14.setText("Stats");
jLabel15.setText(".....................................................................");
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(getContentPane());
getContentPane().setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(javax.swing.GroupLayout.Alignment.TRAILING, layout.createSequentialGroup()
.addGap(0, 0, Short.MAX_VALUE)
.addComponent(jLabel15)
.addGap(72, 72, 72))
.addGroup(javax.swing.GroupLayout.Alignment.TRAILING, layout.createSequentialGroup()
.addGap(53, 60, Short.MAX_VALUE)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(javax.swing.GroupLayout.Alignment.TRAILING, layout.createSequentialGroup()
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jPanel1, javax.swing.GroupLayout.Alignment.TRAILING, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGroup(javax.swing.GroupLayout.Alignment.TRAILING, layout.createSequentialGroup()
.addComponent(jLabel14)
.addGap(141, 141, 141))
.addGroup(layout.createSequentialGroup()
.addGap(24, 24, 24)
.addComponent(jTextField1, javax.swing.GroupLayout.PREFERRED_SIZE, 166, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jButton1)))
.addGap(48, 48, 48))
.addGroup(javax.swing.GroupLayout.Alignment.TRAILING, layout.createSequentialGroup()
.addComponent(jLabel1)
.addGap(110, 110, 110))))
);
layout.setVerticalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addGap(12, 12, 12)
.addComponent(jLabel1)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(jButton1)
.addComponent(jTextField1, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel15)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED, 8, Short.MAX_VALUE)
.addComponent(jLabel14)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jPanel1, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(18, 18, 18))
);
pack();
}// </editor-fold>//GEN-END:initComponents
private boolean isPalindrome(String word) {
StringBuffer userWord = new StringBuffer(); // StringBuffer to hold the user entered word
StringBuffer backwards;// StringBuffer to hold reversed user entered word
//Loop to get rid of non letters and whitespace
for (int i = 0; i < word.length(); i++) {
char goodch = word.charAt(i);
if (Character.isLetterOrDigit(goodch)) {
userWord.append(goodch);
}
}
String normal = userWord.toString();//convert original StringBuffer to String
backwards = userWord.reverse();//reverse userWord and assign to backwards String
String backward = backwards.toString();// convert backwards StringBuffer to String
int n = normal.compareToIgnoreCase(backward);//compare original String to reversed String and ignore the case
if (n == 0) {
return true;// Original String is the same as reversed String, Is Palindrome
} else {
return false;// Original String is NOT the same as reversed String, Is Not Palindrome
}
}
private int[] stringStats(String userWord) {// Method to count characters
int stats[] = new int[6];// array to hold count
for (int i = 0; i < userWord.length(); i++) {//Loop to go through the word, determine which character, then add to the count
Character ch = userWord.charAt(i);
if (Character.isLetter(ch)) {// count for letters
stats[0]++;
}
if (Character.isDigit(ch)) {// count for numbers
stats[1]++;
}
if (Character.isISOControl(ch)) {//count for controls
stats[2]++;
}
if (Character.isUpperCase(ch)) {// count for uppercase
stats[3]++;
}
if (Character.isLowerCase(ch)) {// count for lower case
stats[4]++;
}
if (Character.isWhitespace(ch)) {// count for white space
stats[5]++;
}
}
return stats;
}
private void jButton1ActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_jButton1ActionPerformed
String userWord = jTextField1.getText();// get user entered text from text field and initialize to String userWord
if (isPalindrome(userWord)) { // calling isPalindrome method, if true sets text field to green and displays message
jTextField1.setBackground(Color.green);
jLabel1.setText("It is a Palindrome!");
} else {
jTextField1.setBackground(Color.red);// if not true sets text field to red and displays message
jLabel1.setText("It is NOT a Palindrome!");
}
int stats[] = stringStats(userWord);
String statString[] = new String[6];
for (int i = 0; i < stats.length; i++) {
statString[i] = String.valueOf(stats[i]);
}
// Set the test of each label to the corrosponding counts
jLabel8.setText(statString[0]);
jLabel9.setText(statString[1]);
jLabel10.setText(statString[2]);
jLabel11.setText(statString[3]);
jLabel12.setText(statString[4]);
jLabel13.setText(statString[5]);
}//GEN-LAST:event_jButton1ActionPerformed
/**
* @param args the command line arguments
*/
public static void main(String args[]) {
/* Set the Nimbus look and feel */
//<editor-fold defaultstate="collapsed" desc=" Look and feel setting code (optional) ">
/* If Nimbus (introduced in Java SE 6) is not available, stay with the default look and feel.
* For details see http://download.oracle.com/javase/tutorial/uiswing/lookandfeel/plaf.html
*/
try {
for (javax.swing.UIManager.LookAndFeelInfo info : javax.swing.UIManager.getInstalledLookAndFeels()) {
if ("Nimbus".equals(info.getName())) {
javax.swing.UIManager.setLookAndFeel(info.getClassName());
break;
}
}
} catch (ClassNotFoundException ex) {
java.util.logging.Logger.getLogger(PalindromeChecker.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (InstantiationException ex) {
java.util.logging.Logger.getLogger(PalindromeChecker.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (IllegalAccessException ex) {
java.util.logging.Logger.getLogger(PalindromeChecker.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (javax.swing.UnsupportedLookAndFeelException ex) {
java.util.logging.Logger.getLogger(PalindromeChecker.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
}
//</editor-fold>
/* Create and display the form */
java.awt.EventQueue.invokeLater(new Runnable() {
public void run() {
new PalindromeChecker().setVisible(true);
}
});
}
// Variables declaration - do not modify//GEN-BEGIN:variables
private javax.swing.JButton jButton1;
private javax.swing.JLabel jLabel1;
private javax.swing.JLabel jLabel10;
private javax.swing.JLabel jLabel11;
private javax.swing.JLabel jLabel12;
private javax.swing.JLabel jLabel13;
private javax.swing.JLabel jLabel14;
private javax.swing.JLabel jLabel15;
private javax.swing.JLabel jLabel2;
private javax.swing.JLabel jLabel3;
private javax.swing.JLabel jLabel4;
private javax.swing.JLabel jLabel5;
private javax.swing.JLabel jLabel6;
private javax.swing.JLabel jLabel7;
private javax.swing.JLabel jLabel8;
private javax.swing.JLabel jLabel9;
private javax.swing.JPanel jPanel1;
private javax.swing.JTextField jTextField1;
// End of variables declaration//GEN-END:variables
}