This application creates an interface file and two classes which are used to represent two types of employees. Using the superclass of Employee and the interface of Payroll
you will create two subclasses Hourly and Commission that will be used to calculate their biweekly pay amount.
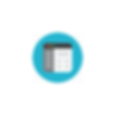
package assignments;
import javax.swing.JOptionPane;
/**
*
* @author danielwevers
*/
// Creating a class "Commission" and inherits constructor and methodsparent class "Employee" and Implements the "Payroll" interface
public class Commission extends Employee implements Payroll{
private double commRate;// Variable attribute for commision pay rate
private double salesWeek1;// Variable attribute for total sales in week 1
private double salesWeek2;// Variable attribute for total sales in week 2
// Constructor for "Commission" class.
public Commission(String id, String name, double commRate) {
super(id,name);//Inherits "id" and "name" from the super class of "Employee"
this.commRate = commRate;// Assigns the "commRate" global attribute to the "commRate" local attribute for "Commission" constructor
}
/* Creating a Commission class method "setPayPeriodDetails" to override the "setPayPeriodDetails"
interface method when Hourly action is performed */
@Override
public void setPayPeriodDetails() {
/* Input dialog box for user to enter total sales in week one.
Parses the String to a Double and stores it in "salesWeek1" object */
salesWeek1 = Double.parseDouble(JOptionPane.showInputDialog("Enter total sales in week 1"));
/* Input dialog box for user to enter total sales in week two.
Parses the String to a Double and stores it in "salesWeek2" object */
salesWeek2 = Double.parseDouble(JOptionPane.showInputDialog("Enter total sales in week 2"));
}
/* Creating a Commission class method "calculatePay()" to override the "calculatePay()"
interface method when Commission action is performed */
@Override
public double calculatePay(){
salesWeek1 = (salesWeek1 * (commRate/100));// Commission calculation for week 1
salesWeek2 = (salesWeek2 * (commRate/100));// Commission calculation for week 2
return salesWeek1 + salesWeek2; // method returns total commission for 2 week period.
}
/* Creating a Commission class method "toString()" to override the "toString()"
interface method when Commission action is performed */
@Override
public String toString(){
/* Method returns the super class "Commission" the Strings "name"
and "id" as well as the return value of the "calculatePay()" method. */
return super.toString() + "\n" + "ID: "+ getId() + "\n" + "Commission Amount: $" + calculatePay();
}
}
package assignments;
import javax.swing.JOptionPane;
/**
*
* @author danielwevers
*/
// Creating a class "Hourly" inherits constructor and methods from parent class "Employee" and Implements the "Payroll" interface
public class Hourly extends Employee implements Payroll{
private double rate; // Variable attribute for hourly pay rate
private double hoursWorkedWeek1; // Variable attribute for number of hours worked in week 1
private double hoursWorkedWeek2;// Variable attribute for number of hours worked in week 2
// Constructor for "Hourly" class.
public Hourly(String id, String name, double rate){
super(id, name);//Inherits "id" and "name" from the super class of "Employee"
this.rate = rate;// Assigns the "rate" global attribute to the "rate" local attribute for "Hourly" constructor
}
/* Creating a class method "setPayPeriodDetails" to override the "setPayPeriodDetails"
interface method when Hourly action is performed */
@Override
public void setPayPeriodDetails() {
/*Input dialog box for user to enter hours worked in week one.
Parses the String to a Double and stores it in "hoursWorkedWeek1" object */
hoursWorkedWeek1 = Double.parseDouble(JOptionPane.showInputDialog("Enter number of hours worked in week 1"));
/*Input dialog box for user to enter hours worked in week two.
Parses the String to a Double and storess it in "hoursWorkedWeek2" object */
hoursWorkedWeek2 = Double.parseDouble(JOptionPane.showInputDialog("Enter number of hours worked in week 2"));
}
/* Creating a class method "calculatePay()" to override the "calculatePay()"
interface method when Hourly action is performed */
@Override
public double calculatePay() {
double week1pay, week2pay;// Variable attribute to hold total week pay.
double overtimeWeek1, overtimeWeek2;// Variable attribute to hold overtime hours over 40.
/* If statement to determine if employee worked overtime in week 1.
If true, pay is calculate with overtime rate of 1.5. If false, pay is calculated normally
*/
if (hoursWorkedWeek1 > 40.0){
overtimeWeek1 = hoursWorkedWeek1 - 40;
hoursWorkedWeek1 = 40;
week1pay = (overtimeWeek1 * (rate *1.5)) + (hoursWorkedWeek1 * rate);
}else week1pay = rate * hoursWorkedWeek1;
/* If statement to determine if employee worked overtime in week 2.
If true, hours over 40 are calculate with overtime rate of 1.5, plus the regular time at the regualar rate.
If false, pay is calculated normally
*/
if (hoursWorkedWeek2 > 40.0){
overtimeWeek2 = hoursWorkedWeek2 - 40;
hoursWorkedWeek2 = 40;
week2pay = (overtimeWeek2 * (rate * 1.5)) + (hoursWorkedWeek2 * rate);
}else week2pay = rate * hoursWorkedWeek2;
return week1pay + week2pay; // method returns total pay for 2 week period.
}
/* Creating a class method "toString()" to override the "toString()"
interface method when Hourly action is performed */
@Override
public String toString(){
/* Method returns the super class "Hourly" the Strings "name"
and "id" as well as the return value of the "calculatePay()" method. */
return super.toString() + "\n" + "ID: "+ getId() + "\n" + "Payment Amount: $" + calculatePay();
}
}
package assignments;
/**
*
* @author danielwevers
*/
// Creating a public interface for multiple classes to implement
public interface Payroll {
public void setPayPeriodDetails(); // Method to set pay period details
public double calculatePay();// Method to calculate Pay
}